Shopify app frontendsÂ
Gadget offers a comprehensive toolkit for quickly constructing frontends for Shopify Apps. When connecting a Gadget app to Shopify, it automatically includes a basic, hosted frontend powered by Vite. This frontend comes preloaded with support for OAuth, Shopify's Polaris design system, multi-tenant data security, and integration with Shopify's App Bridge for embedded applications. You have the flexibility to customize this frontend according to your preferences, or you can create an entirely new external frontend from scratch using Gadget's React packages.
Frontends for Shopify apps interact with Gadget backends through the GraphQL API specific to your Gadget app, along with the corresponding JavaScript client for your application. To work with Gadget from a Shopify app frontend, there are several npm packages that are essential:
Package | Description | Available from |
---|---|---|
@shopify/app-bridge | Shopify's React package for embedding React applications within the Shopify Admin | npm |
@gadget-client/example-app | The JS client for your specific Gadget application | Gadget NPM registry |
@gadgetinc/react | The Gadget React bindings library, providing React hooks for making API calls | npm |
@gadgetinc/react-shopify-app-bridge | The Gadget Shopify wrapper library for Shopify Embedded App setup and authentication | npm |
Gadget installs these packages into your Gadget-hosted frontend automatically, but if you're building an external frontend you must install them yourself.
Once you've set up your Shopify connection, your Gadget app will have a built-in frontend ready for construction in your app's frontend
folder. This frontend can access data from your backend, including both models synced from Shopify and models created within your application. By default, your app uses React and Shopify's standard @shopify/app-bridge-react
library, so Shopify's normal helpers for navigation and data fetching are ready for use as well.
Gadget frontends for Shopify include Shopify's design system Polaris via the @shopify/polaris
package as well, so your app is compliant with Shopify's App Store guidelines out of the box.
If you'd like to build your frontend outside of Gadget, refer to the external frontends guide.
Reading data from your backendÂ
To facilitate easy access to data from your Gadget application, including both the non-rate-limited copies of Shopify models and your custom models, you can leverage the @gadgetinc/react hooks library in your frontend. This library provides a set of hooks, such as useFindOne
, useFindMany
, and useFindFirst
, specifically designed for fetching data from your Gadget app.
When utilizing these hooks, each one returns an object that includes the requested data, the current fetching state, and an error object if any error occurred during the data retrieval process. Additionally, the returned object includes a refetch function that allows you to refresh the data if needed.
By using the provided hooks from the @gadgetinc/react
library, you can easily fetch and manage data from your Gadget app within your frontend code. These hooks simplify the process of data retrieval, provide relevant states and error handling, and offer a convenient mechanism for refreshing the data when necessary.
For example, if you have the Shopify Product model enabled in your Connection, we can fetch product records in a variety of ways:
jsx1// fetch one product by id2const [{ data, fetching, error }, refetch] = useFindOne(api.shopifyProduct, 10);34// fetch the first 10 products5const [{ data, fetching, error }, refetch] = useFindMany(api.shopifyProduct, { first: 10 });67// fetch the first product with the title field equal to "Socks", throw if it isn't found8const [{ data, fetching, error }, refetch] = useFindFirst(api.shopifyProduct, { where: { title: "Socks" } });910// fetch the first product with the title field equal to "Socks", return null if it isn't found11const [{ data, fetching, error }, refetch] = useMaybeFindFirst(api.shopifyProduct, { where: { title: "Socks" } });
Data from other models that you've created in your application is accessed the same way. For example, if we're building a free shipping banner app, you might create a Banner model that stores details about each shipping banner created. We can fetch banner records with the same React hooks:
jsx// fetch one banner by idconst [{ data, fetching, error }, refetch] = useFindOne(api.banner, 10);// fetch the first 10 bannersconst [{ data, fetching, error }, refetch] = useFindMany(api.banner, { first: 10 });
Each of these hooks must be wrapped in a React component to render. For example, we can use useFindMany
to display a list of products in a component:
jsx1import { useFindMany } from "@gadgetinc/react";2import { api } from "../api";34export const ProductsList = (props) => {5 const [{ data, fetching, error }, _refetch] = useFindMany(api.shopifyProduct, { first: 10 });67 if (fetching) {8 return <div>Loading...</div>;9 }1011 if (error) {12 return <div>Error: {error.message}</div>;13 }1415 return <ul>16 {data.map((product) => <li key={product.id}>{product.title}</li>)}17 </li>18}
For more on reading data in the frontend, see the building frontends guide and the @gadgetinc/react reference.
Shopify permissionsÂ
Gadget incorporates multi-tenant data permissions as the default configuration for your application. By default, an app loaded for a specific Shopify shop can only access data within that particular shop and is not authorized to access data from other shops. This default setup utilizes the Shopify App User role for enforcing these permissions, and you have the flexibility to customize the permissions for this role on the Roles & Permissions page.
In Gadget, multi-tenant data permissions are automatically enforced for Shopify models. However, access to your own models is not automatically granted. To enable frontend access to your models, you need to assign permissions to the Shopify App User role for each model you wish to make accessible. For instance, if you want to access a model named Banner, you can navigate to the Roles & Permissions screen and select the Read permission checkbox for the Banner model.
For further guidance and details on managing access control, refer to the access control guide, which provides comprehensive information on configuring and customizing data permissions in Gadget.
Writing data back to your backendÂ
Shopify app frontends can use the useActionForm
, useAction
and useGlobalAction
hooks from the @gadgetinc/react
hooks library to write data back to your database.
To update data within Shopify, you must make an API call to Shopify directly from your backend. See the Calling Shopify API section for more information.
To write data back to your database for models you've created, or fields you've added to Shopify Models, use useActionForm
, useAction
or useGlobalAction
hook in a React component.
For example, if we create a new model within our app called banner
, we can use the useActionForm
hook to create a form for new banner records:
jsx1import { useActionForm } from "@gadgetinc/react";2import { api } from "../api";34export const CreateBannerForm = (props) => {5 const {6 register,7 submit,8 formState: { isSubmitting },9 error,10 } = useActionForm(api.banner.create);1112 if (isSubmitting) {13 return <div>Saving...</div>;14 }1516 if (error) {17 return <div>Error: {error.message}</div>;18 }1920 return (21 <form onSubmit={submit}>22 <label>Message</label>23 <textarea {...register("message")}>{message}</textarea>24 <input type="submit" />25 </form>26 );27};
For more details on the useActionForm
hook, see the @gadgetinc/react
reference, and see the Building Frontends guide for more examples.
Calling Global ActionsÂ
Shopify app frontends can call your backend's Global Actions with the useGlobalAction
hook. See the Building Frontends guide for more information.
Calling HTTP routesÂ
Shopify app frontends can call your backend's HTTP Routes with api.fetch
, or any other HTTP client for React. See the Building Frontends guide for more information.
If your HTTP routes require authentication, or need to access the connections
object server side, you must ensure you pass the correct
authentication headers to your HTTP route from your frontend. You can do this automatically by using api.fetch
instead of the built-in
browser fetch
.
For more information, see the Building Frontends
guide.
Calling the Shopify APIÂ
In Gadget, it is generally recommended to read data through Gadget's API, as Gadget's API does not impose the same access restrictions as Shopify's API. Unlike Shopify's API, your Gadget app's API is not rate-limited, allowing you to fetch data without the need for meticulous request management.
However, if you require access to data that your Gadget app doesn't sync or if you need to retrieve data that Gadget doesn't have direct access to, you can utilize Shopify's API directly.
To make requests to Shopify's Admin API, it is best to initiate the calls from the backend of your Gadget app's API. This involves making a request from your frontend application to your Gadget backend, which in turn makes the necessary calls to Shopify's API. The results are then returned from your Gadget backend to your frontend application.
Calling Shopify within actionsÂ
Code within a model actions or global actions can make API calls to Shopify using the connections.shopify.current
Shopify API client Gadget provides.
For example, if we want to create a product from the frontend of our Gadget application, we can create an global action that calls the Shopify API:
First, we create a new global action called createProduct.js
within the api/actions
folder in Gadget.
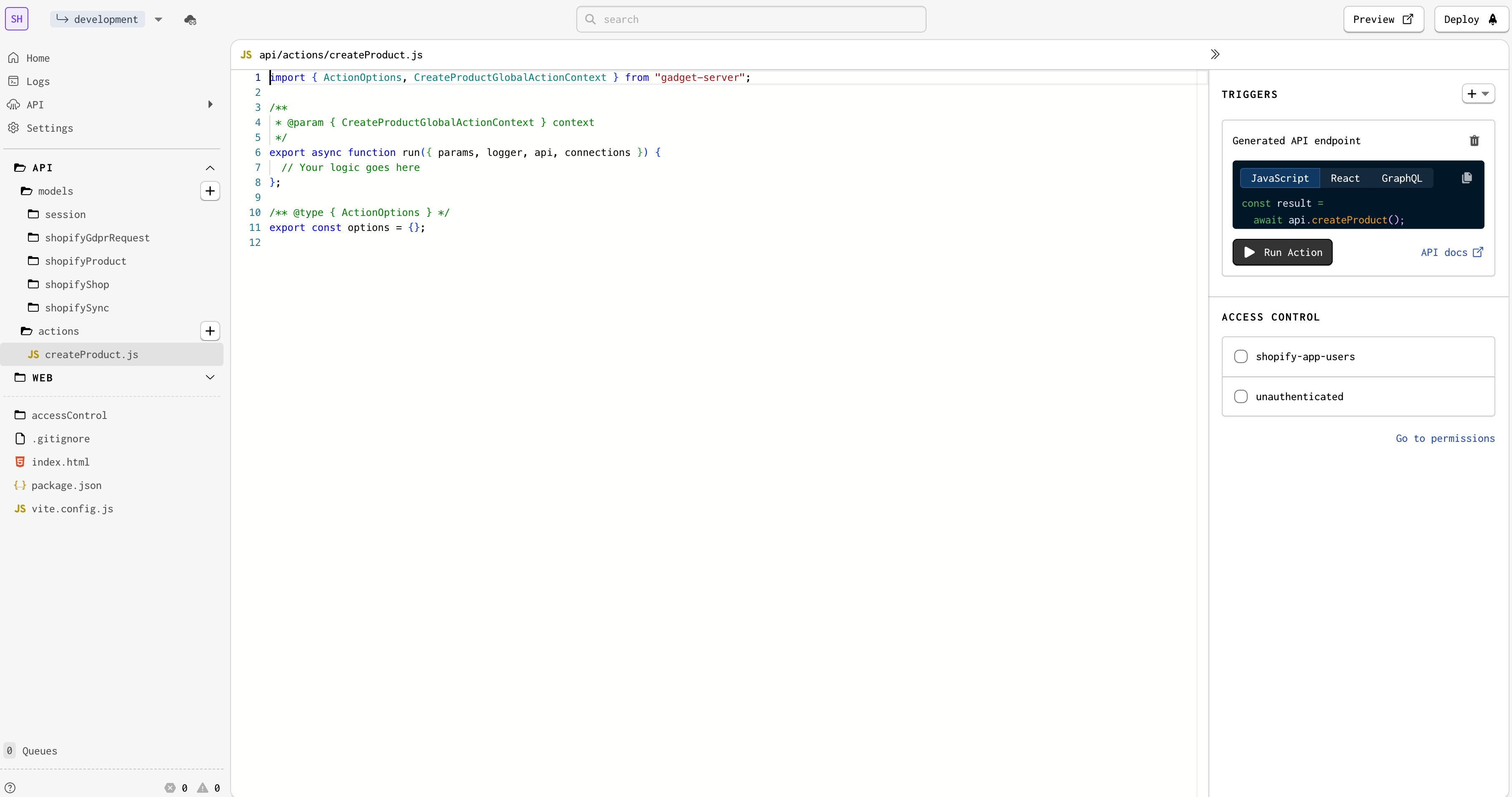
Then, we can add the following code to the global action:
api/actions/createProduct.jsJavaScript1export async function run({ scope, logger, params, connections }) {2 const shopify = connections.shopify.current;3 const product = await shopify.product.create({4 title: "test 123",5 });6 logger.info({ product }, "created new product in shopify");7 scope.result = product;8}
This action uses Gadget's connections
object to access the Shopify API client, and then calls the product.create
method to create a new product in Shopify.
We can then call this global action from our Shopify frontend with the useGlobalAction
React hook. For example, we can call this action when a button is clicked in the app:
web/components/CreateProductButton.jsjsx1import { useGlobalAction } from "@gadgetinc/react";2import { api } from "../api";34export const CreateProductButton = (props) => {5 const [{ data, fetching, error }, act] = useGlobalAction(api.createProduct);67 return (8 <button disabled={fetching} onClick={() => void act()}>9 Create Product10 </button>11 );12};
Gadget's synced Shopify models are one-way synced out of Shopify. You can't call the api.shopifyProduct.create
action from the frontend
to create a product, as that would put your Gadget app out of sync with Shopify. You must use the Shopify API to create resources that
Shopify owns.
Calling Shopify in HTTP RoutesÂ
Route code within HTTP Routes can access the Shopify API using request.connections.shopify
to create a Shopify API client object, similarly to Actions or Global Actions.
For example, an HTTP route could return an up-to-date list of products from the Shopify API:
JavaScript1export default async function route({ request, reply }) {2 const shopify = request.connections.shopify.current;3 const products = await shopify.product.list();4 reply.send({5 products,6 });7}
If you're accessing the connections
object server side, you must ensure you pass the correct authentication headers to your HTTP route
from your frontend. You can do this automatically by using api.fetch
in the browser instead of the built-in browser fetch
.
For more information, see the Building Frontends
guide.
Standalone Shopify AppsÂ
With a standalone Shopify app, you'll need to handle authentication and tenancy yourself as there is no Shopify session token to derive this from.
If your Shopify app is not an embedded app, you can still use the Gadget frontends to build your app. To do so, you will need to set the type
prop on GadgetProvider
to AppType.Standalone
.
<GadgetProvider type={AppType.Standalone} shopifyApiKey={window.gadgetConfig.apiKeys.shopify} api={api} router={appBridgeRouter}><AuthenticatedApp /></GadgetProvider>
Next is to update your routes/shopify/GET-install.js
route file to redirect users back to your frontend instead of the Shopify admin.
1// replace the following2// if (embedded) {3// return await reply.redirect("/?" + new URLSearchParams(query).toString());4// } else {5// const host = Buffer.from(base64Host, 'base64').toString('ascii');6// return await reply.redirect(`https://${host}/apps/${apiKey}`);7// }89return await reply.redirect("/");
This will ensure that once a shop install has completed users will be redirected to your frontend.
Shopify frontend security requirementsÂ
Shopify requires app developers to meet a set of important security requirements when building embedded apps. Gadget meets each security requirement out of the box:
- Gadget apps are always served over HTTPS
- Gadget apps run behind a production-grade firewall and only expose the necessary services
- Gadget apps use robust, multi-tenant authentication that limits access to data to the current shop (see the access control guide)
- Gadget sets up GDPR webhook listeners automatically for Shopify apps (see the GDPR docs)
- Gadget uses Shopfiy's Session Token authentication mechanism for authenticating the frontend to the backend.
- Gadget automatically sets the
Content-Security-Policy
header necessary for Shopify's IFrame protection when serving Shopify apps (see Shopify's security docs)
If you have any questions about the security posture of Gadget applications for Shopify, please join us in our Discord to discuss!
ReferenceÂ
The Provider
Â
The @gadgetinc/react-shopify-app-bridge
library handles authentication of your embedded app via the Provider
component. This provider has two main benefits - it handles authentication and the series of redirects required to complete an embedded app OAuth flow in Shopify, and it handles retrieving a Shopify session token from the App Bridge and passing it along to Gadget for authenticated calls.
The Provider handles these key tasks automatically:
- Starts the OAuth process with new users of the application using Gadget, escaping Shopify's iframe if necessary
- Establishes an iframe-safe secure session with the Gadget backend using Shopify's Session Token authentication scheme
- Sets up the correct React context for making backend calls to Gadget using
@gadgetinc/react
The Provider
has the following required props:
export interface ProviderProps {type: AppType; // 'AppType.Embedded' or 'AppType.Standalone'shopifyApiKey: string; // the API key from your Shopify app in the partner dashboard that is used with the Shopify App Bridgeapi: string; // the API client created using your Gadget application}
The Gadget provider will handle detecting if your app is being rendered in an embedded context and redirect the user through Shopify's OAuth flow if necessary.
The useGadget
React hookÂ
The Provider
handles initializing the App Bridge for us. Now we can build our application component and use the initialized instance of App Bridge via the appBridge
key returned from the embedded React hook useGadget
.
useGadget
provides the following properties:
1export interface useGadget {2 isAuthenticated: boolean; // 'true' if the user has completed a successful OAuth flow3 isEmbedded: boolean; // 'true' if the app is running in an embedded context4 isRootFrameRequest: boolean; // 'true' if a user is viewing a "type: AppType.Embedded" app in a non-embedded context, for example, accessing the app at a hosted Vercel domain5 loading: boolean; // 'true' if the OAuth flow is in process6 appBridge: AppBridge; // a ready-to-use app bridge from Shopify, you can also use the traditional useAppBridge hook in your components to retrieve it.7}
useGadget
exampleÂ
The following example renders a ProductManager component that makes use of Shopify App Bridge components and is ready to be embedded in a Shopify Admin page.
1import { useAction, useFindMany } from "@gadgetinc/react";2import { useGadget } from "@gadgetinc/react-shopify-app-bridge";3import { Button, Redirect, TitleBar } from "@shopify/app-bridge/actions";4import { api } from "./api.ts";56function ProductManager() {7 const { loading, appBridge, isRootFrameRequest } = useGadget();8 const [_, deleteProduct] = useAction(api.shopifyProduct.delete);9 const [{ data, fetching, error }, refresh] = useFindMany(api.shopifyProduct);1011 if (error) return <>Error: {error.toString()}</>;12 if (fetching) return <>Fetching...</>;13 if (!data) return <>No widgets found</>;1415 // Set up a title bar for the embedded app16 const breadcrumb = Button.create(appBridge, { label: "My breadcrumb" });17 breadcrumb.subscribe(Button.Action.CLICK, () => {18 appBridge.dispatch(Redirect.toApp({ path: "/breadcrumb-link" }));19 });2021 const titleBarOptions = {22 title: "My page title",23 breadcrumbs: breadcrumb,24 };25 TitleBar.create(appBridge, titleBarOptions);2627 return (28 <>29 {loading && <span>Loading...</span>}30 {isRootFrameRequest && (31 <span>App can only be viewed in the Shopify Admin!</span>32 )}33 {!loading &&34 !isRootFrameRequest &&35 data.map((widget, i) => (36 <button37 key={i}38 onClick={(event) => {39 event.preventDefault();40 void deleteProduct({ id: widget.id }).then(() => refresh());41 }}42 >43 Delete {widget.title}44 </button>45 ))}46 </>47 );48}
GraphQL queriesÂ
When developing embedded Shopify apps, it is possible that the installed scopes of a Shop may not align with the required scopes in your Gadget app's connection. In such cases, it becomes necessary to re-authenticate with Shopify in order to obtain the updated scopes. To determine if re-authentication is required and gather information about missing scopes, you can execute the following GraphQL query using the app client provided to the Provider
:
1query {2 shopifyConnection {3 requiresReauthentication4 missingScopes5 }6}
Session model managementÂ
The Shopify Connection in Gadget automatically manages records of the backend Session model when using @gadgetinc/react-shopify-app-bridge
. When a merchant first loads up the frontend application, the <Provider/>
will retrieve a Shopify Session Token from Shopify's API, and pass it to your Gadget backend application. The Gadget Shopify Connection will then validate this token. If valid, the connection will provision a new record of the Session model with the correct shopId
field set up. This session is then passed to all your backend application's model filters and is available within Action code snippets.
Embedded app examplesÂ
Want to see an example of an embedded Shopify app built using Gadget?
Check out some of our example apps on GitHub, including:
External Frontends with the Shopify CLIÂ
If the built-in Gadget frontend doesn't work for you, you can use the frontend generated by the Shopify CLI as an external frontend for your Gadget backend application. This lets you still take advantage of Gadget's OAuth handling, scalable backend database, and robust webhook processing while using the frontend generated by the Shopify CLI.
Using the Shopify CLI frontendÂ
To use Shopify's generated frontend as an external frontend, you will need to make a few changes to the code generated by the Shopify CLI. Instead of deleting the web
folder from your repository after generating a CLI app, keep it in place, and follow these steps:
- Update
web/index.js
to not implement Shopify OAuth, as Gadget handles OAuth and syncing data from the Shopify API. Instead,web/index.js
just needs to serve the frontend application with the correct security headers for Shopify.
Replace the contents of web/index.js
with the following:
web/index.jsJavaScript1// @ts-check2import { join } from "path";3import * as fs from "fs";4import express from "express";5import serveStatic from "serve-static";67const __dirname = new URL(".", import.meta.url).pathname;89const PORT = parseInt(process.env["BACKEND_PORT"] || process.env["PORT"], 10);10const STATIC_PATH =11 process.env["NODE_ENV"] === "production"12 ? `${__dirname}/frontend/dist`13 : `${__dirname}/frontend/`;1415const app = express();1617// return Shopify's required iframe embedding headers for all requests18app.use((req, res, next) => {19 const shop = req.query.shop;20 if (shop) {21 res.setHeader(22 "Content-Security-Policy",23 `frame-ancestors https://${shop} https://admin.shopify.com;`24 );25 }26 next();27});2829// serve any static assets built by vite in the frontend folder30app.use(serveStatic(STATIC_PATH, { index: false }));3132// serve the client side app for all routes, allowing it to pick which page to render33app.use("/*", async (_req, res, _next) => {34 return res35 .status(200)36 .set("Content-Type", "text/html")37 .send(fs.readFileSync(join(STATIC_PATH, "index.html")));38});3940app.listen(PORT);
- You can then delete the other example code that
@shopify/cli
created in theweb/
directory when it created your app if you like by running the following command in your app's root directory
terminalrm -f web/shopify.js web/product-creator.js web/gdpr.js
Finally, we can set up our Gadget Client and use the Provider to handle OAuth for our embedded app.
You need to install your Gadget dependencies in the web/frontend
directory of your Shopify CLI application! Change into this directory before running the following commands:
terminalcd web/frontend
- Install
local-ssl-proxy
in theweb/frontend
directory
npm install local-ssl-proxy
yarn add local-ssl-proxy
- Update the
dev
script inweb/frontend/package.json
tovite & local-ssl-proxy --source 443 --target 3005
.
1{2 // ...3 "scripts": {4 "build": "vite build",5 "dev": "vite & local-ssl-proxy --source 443 --target 3005",6 "coverage": "vitest run --coverage"7 }8}
If you are working with Windows, the dev
command above will not work. You will need to split it up into two separate commands and run
them separately. For example, "dev": "vite"
and "dev-proxy": "local-ssl-proxy --source 443 --target 3005"
.
This allows us to use our local frontend when doing development inside Shopify's admin, which uses HTTPS.
- Replace your
web/frontend/vite.config
file with the following code:
web/frontend/vite.config.jsJavaScript1import { defineConfig } from "vite";2import { dirname } from "path";3import { fileURLToPath } from "url";4import react from "@vitejs/plugin-react";56if (7 process.env["npm_lifecycle_event"] === "build" &&8 !process.env["CI"] &&9 !process.env["SHOPIFY_API_KEY"]10) {11 console.warn(12 "\nBuilding the frontend app without an API key. The frontend build will not run without an API key. Set the SHOPIFY_API_KEY environment variable when running the build command.\n"13 );14}1516const host = "localhost";17const port = 3005;1819export default defineConfig({20 root: dirname(fileURLToPath(import.meta.url)),21 plugins: [react()],22 define: {23 "process.env": JSON.stringify({24 SHOPIFY_API_KEY: process.env["SHOPIFY_API_KEY"],25 }),26 },27 resolve: {28 preserveSymlinks: true,29 },30 server: {31 host: host,32 port: port,33 hmr: {34 protocol: "ws",35 host: host,36 port: port,37 clientPort: port,38 },39 },40});
If you wish to change the port for your local server, make sure to modify both the port variable in web/frontend/vite.config
and the
target at the end of the dev
script in the web/frontend/package.json
. Note that the ports must be the same for the proxy to function
correctly.
Shopify CLI apps using Gadget don't need to use ngrok and instead run at https://localhost
. This vite config keeps vite's hot module reloading functionality working quickly without using ngrok which is faster and more reliable.
- You need to register the Gadget NPM registry for the
@gadget-client
package scope:
web/frontendnpm config set @gadget-client:registry https://registry.gadget.dev/npm
- The following npm modules are required when creating an app that will be embedded in the Shopify Admin:
npm install @gadgetinc/react @gadgetinc/react-shopify-app-bridge @gadget-client/example-app
yarn add @gadgetinc/react @gadgetinc/react-shopify-app-bridge @gadget-client/example-app
Make sure to replace `example-app` with your app's package name!
- To deploy your frontend using hosting platforms such as Vercel, Heroku or Netlify, you will need to add a new file
web/frontend/.npmrc
to help point to the Gadget registry.
@gadget-client:registry=https://registry.gadget.dev/npm
- The next step is to set up your Gadget client in the application. You can use this client to make requests to your Gadget application. You can create a new file in your project, and add the following code:
import { Client } from "@gadget-client/example-app";export const api = new Client();
- Now you need to set up the Provider in
web/frontend/App.jsx
. We can also use theuseGadget
hook to ensure we are authenticated before we make requests using the API. Here is a small snippet as an example:
web/frontend/App.jsxJavaScript1import {2 AppType,3 Provider as GadgetProvider,4 useGadget,5} from "@gadgetinc/react-shopify-app-bridge";6import { api } from "./api";78import { PolarisProvider } from "./components";910/**11 Gadget's Provider takes care of App Bridge authentication, you do not need Shopify's default AppBridgeProvider.12*/13export default function App() {14 return (15 <GadgetProvider16 type={AppType.Embedded}17 shopifyApiKey={process.env["SHOPIFY_API_KEY"]}18 api={api}19 >20 <PolarisProvider>21 <EmbeddedApp />22 </PolarisProvider>23 </GadgetProvider>24 );25}2627// This is where we make sure we have auth'd with AppBridge28// Once we have authenticated, we can render our app!29// Feel free to use the default page navigation that Shopify's CLI sets up for you30// example here - https://github.com/gadget-inc/examples/blob/main/packages/shopify-cli-embedded/web/frontend/App.jsx31function EmbeddedApp() {32 // we use `isAuthenticated` to render pages once the OAuth flow is complete!33 const { isAuthenticated } = useGadget();34 return isAuthenticated ? (35 <span>Hello, world!</span>36 ) : (37 <span>Authenticating...</span>38 );39}
If you are looking for examples of how to use our API client, visit our examples repository.
Next stepsÂ
Once you've updated your Shopify CLI web
folder to function as an external frontend, you can start building your app! See the Shopify connection guide for more information on building apps for Shopify with Gadget.
DeploymentÂ
If using the Shopify CLI's web
folder as the frontend for your application, you'll need to deploy it to a hosting platform. See the External Frontends guide for more information on deploying your frontend elsewhere.
Shopify App Bridge V4 supportÂ
Gadget supports using Shopify's App Bridge V4, which is the first version from Shopify to use their new CDN-based delivery mechanism. Read more about the App Bridge in Shopify's docs.
RequirementsÂ
When using version 4 of Shopify's App Bridge, applications must depend on 3 pieces:
- a
<script/>
tag to import the code for the app bridge from Shopify's CDN (automatically added at runtime by Gadget) - the
@shopify/app-bridge-react
package from npm, version 4 or higher - the
@gadgetinc/react-shopify-app-bridge
package from npm, version0.14
or higher
If you were previously using the @shopify/app-bridge
package from npm, it must be removed.
CDN Script TagÂ
Shopify requires that the basic code for the app bridge is required from their CDN, instead of bundled into your application. This allows Shopify to ship changes to the app bridge like bug fixes and performance improvements without requiring you to redeploy your application. The CDN-based approach is what Shopify endorses, is required for the Built For Shopify badge, and the only version of the app-bridge still receiving updates. Gadget recommends adopting this CDN-based approach for all Shopify applications.
Gadget will automatically insert the <script/>
tag to import the App Bridge from Shopify's CDN when using @gadgetinc/react-shopify-app-bridge
version 0.14
or later.
Upgrade procedureÂ
For existing apps on older versions who wish to upgrade, follow these steps:
- Upgrade the
@shopify/app-bridge-react
package to4.x.x
by installing the latest version. For reference on using terminal commands within Gadget check out our guide here.
Run in the Gadget command paletteyarnyarn upgrade @shopify/app-bridge-react@latest
- Upgrade the
@gadgetinc/react-shopify-app-bridge
package to0.14.1
by installing the latest version.
Run in the Gadget command paletteyarnyarn upgrade @gadgetinc/react-shopify-app-bridge@latest
- Now remove
@shopify/app-bridge
dependency by uninstalling it.
Run in the Gadget command paletteyarnyarn remove @shopify/app-bridge
- Finally follow Shopify's guide here on updating your components and hooks to work with Shopify App Bridge V4.
Resulting codeÂ
Each app's code for working with the new library may need to be different, depending on which hooks are being used. As an example, here's the base code for the App.jsx
file for the new version of the Shopify App Bridge:
Before
web/components/App.jsxJavaScript1import {2 AppType,3 Provider as GadgetProvider,4 useGadget,5} from "@gadgetinc/react-shopify-app-bridge";6import { NavigationMenu } from "@shopify/app-bridge-react";7import { Page, Spinner, Text } from "@shopify/polaris";8import { useEffect, useMemo } from "react";9import {10 Outlet,11 Route,12 RouterProvider,13 createBrowserRouter,14 createRoutesFromElements,15 useLocation,16 useNavigate,17} from "react-router-dom";18import Index from "../routes/index";19import AboutPage from "../routes/about";20import { api } from "../api";2122function Error404() {23 const navigate = useNavigate();24 const location = useLocation();2526 useEffect(() => {27 if (28 location.pathname ===29 new URL(process.env.GADGET_PUBLIC_SHOPIFY_APP_URL).pathname30 )31 return navigate("/", { replace: true });32 }, [location.pathname]);3334 return <div>404 not found</div>;35}3637function App() {38 const router = createBrowserRouter(39 createRoutesFromElements(40 <Route path="/" element={<Layout />}>41 <Route index element={<Index />} />42 <Route path="/about" element={<AboutPage />} />43 <Route path="*" element={<Error404 />} />44 </Route>45 )46 );4748 return (49 <>50 <RouterProvider router={router} />51 </>52 );53}5455function Layout() {56 const navigate = useNavigate();57 const location = useLocation();58 const history = useMemo(59 () => ({ replace: (path) => navigate(path, { replace: true }) }),60 [navigate]61 );6263 const appBridgeRouter = useMemo(64 () => ({65 location,66 history,67 }),68 [location, history]69 );7071 return (72 <GadgetProvider73 type={AppType.Embedded}74 shopifyApiKey={window.gadgetConfig.apiKeys.shopify}75 api={api}76 router={appBridgeRouter}77 >78 <AuthenticatedApp />79 </GadgetProvider>80 );81}8283function AuthenticatedApp() {84 // we use `isAuthenticated` to render pages once the OAuth flow is complete!85 const { isAuthenticated, loading } = useGadget();86 if (loading) {87 return (88 <div89 style={{90 display: "flex",91 justifyContent: "center",92 alignItems: "center",93 height: "100%",94 width: "100%",95 }}96 >97 <Spinner accessibilityLabel="Spinner example" size="large" />98 </div>99 );100 }101 return isAuthenticated ? <EmbeddedApp /> : <UnauthenticatedApp />;102}103104function EmbeddedApp() {105 return (106 <>107 <Outlet />108 <NavigationMenu109 navigationLinks={[110 {111 label: "Shop Information",112 destination: "/",113 },114 {115 label: "About",116 destination: "/about",117 },118 ]}119 />120 </>121 );122}123124function UnauthenticatedApp() {125 return (126 <Page title="App">127 <Text variant="bodyMd" as="p">128 App can only be viewed in the Shopify Admin.129 </Text>130 </Page>131 );132}133134export default App;
After
web/components/App.jsxJavaScript1import {2 AppType,3 Provider as GadgetProvider,4 useGadget,5} from "@gadgetinc/react-shopify-app-bridge";6import { NavMenu } from "@shopify/app-bridge-react";7import { Page, Spinner, Text } from "@shopify/polaris";8import { useEffect, useMemo } from "react";9import {10 Outlet,11 Route,12 RouterProvider,13 createBrowserRouter,14 createRoutesFromElements,15 useLocation,16 useNavigate,17 Link,18} from "react-router-dom";19import Index from "../routes/index";20import AboutPage from "../routes/about";21import { api } from "../api";2223function Error404() {24 const navigate = useNavigate();25 const location = useLocation();2627 useEffect(() => {28 if (29 location.pathname ===30 new URL(process.env.GADGET_PUBLIC_SHOPIFY_APP_URL).pathname31 )32 return navigate("/", { replace: true });33 }, [location.pathname]);3435 return <div>404 not found</div>;36}3738function App() {39 const router = createBrowserRouter(40 createRoutesFromElements(41 <Route path="/" element={<Layout />}>42 <Route index element={<Index />} />43 <Route path="/about" element={<AboutPage />} />44 <Route path="*" element={<Error404 />} />45 </Route>46 )47 );4849 return (50 <>51 <RouterProvider router={router} />52 </>53 );54}5556function Layout() {57 return (58 <GadgetProvider59 type={AppType.Embedded}60 shopifyApiKey={window.gadgetConfig.apiKeys.shopify}61 api={api}62 router={appBridgeRouter}63 >64 <AuthenticatedApp />65 </GadgetProvider>66 );67}6869function AuthenticatedApp() {70 // we use `isAuthenticated` to render pages once the OAuth flow is complete!71 const { isAuthenticated, loading } = useGadget();72 if (loading) {73 return (74 <div75 style={{76 display: "flex",77 justifyContent: "center",78 alignItems: "center",79 height: "100%",80 width: "100%",81 }}82 >83 <Spinner accessibilityLabel="Spinner example" size="large" />84 </div>85 );86 }87 return isAuthenticated ? <EmbeddedApp /> : <UnauthenticatedApp />;88}8990function EmbeddedApp() {91 return (92 <>93 <Outlet />94 <NavMenu>95 <Link to="/" rel="home">96 Shop Information97 </Link>98 <Link to="/about">About</Link>99 </NavMenu>100 </>101 );102}103104function UnauthenticatedApp() {105 return (106 <Page title="App">107 <Text variant="bodyMd" as="p">108 App can only be viewed in the Shopify Admin.109 </Text>110 </Page>111 );112}113114export default App;
If main.jsx
still references BrowserRouter
, it should be removed as it will not be compatible with RouterProvider
in App.jsx